https://nmomos.com/tips/2019/07/05/django-social-auth/
google の oauth を使用したログインを実現する為、
上記urlの google 関連部分を写経。
TODO
参考url
Step1: python仮想環境作成と auth-app-django の install や django project 作成
## 仮想環境 作成
$ cd ~/dev/
$ python3 -m venv venv_social_auth
## 仮想環境 有効化
$ cd venv_social_auth
$ source ./bin/activate
## django や social-auth-app-django の install
$ pip install django==2.2
$ pip install social-auth-app-django
## django project 作成.
## (通常、djangoでは config dir名 = project名となる為
## 一度、config の名前でproject を作成し、後でdir名を変更)
$ django-admin startproject config
$ mv config social_auth
※「python manage.py startapp ~」のように通常、project内に
アプリを作成しますが、今回は極小規模ですので、これを行いません。
Step2: auth-app-django install 後の設定
$ vi config/setting.py
ALLOWED_HOSTS = ['*'] ## CHANGE
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'social_django', ## ADD
]
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
'social_django.middleware.SocialAuthExceptionMiddleware', ## ADD
]
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR, 'templates')], ## CHANGE
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
'social_django.context_processors.backends', ## ADD
'social_django.context_processors.login_redirect', ## ADD
],
},
},
]
AUTHENTICATION_BACKENDS = ( ## ADD
'social_core.backends.open_id.OpenIdAuth', ## ADD
'social_core.backends.google.GoogleOpenId', ## ADD
'social_core.backends.google.GoogleOAuth2', ## ADD
'social_core.backends.github.GithubOAuth2', ## ADD
'social_core.backends.twitter.TwitterOAuth', ## ADD
'social_core.backends.facebook.FacebookOAuth2', ## ADD
'django.contrib.auth.backends.ModelBackend', ## ADD
) ## ADD
LOGIN_URL = 'login' ## ADD
LOGIN_REDIRECT_URL = 'home' ## ADD
$ vi config/urls.py
from django.conf.urls import include ## ADD
from django.contrib.auth import views as auth_views ## ADD
from . import views ## ADD
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index, name='index'), ## ADD
path('login/', auth_views.LoginView.as_view(), name='login'), ## ADD
path('logout/', auth_views.LogoutView.as_view(), name='logout'), ## ADD
path('oauth/', include('social_django.urls', namespace='social')), ## ADD
path('accounts/profile/', views.index, name='index') ## ADD
]
Step3: auth-app-django install 後の db migrate
social-app-django は migrate 情報を持っている為、
事前の「python manage.py makemigrations」実行は不要です
$ python manage.py migrate
Step4: Goolge Developers Console で クライアント ID/シークレット取得
以降では、Googleのproject作成後における認証情報設定等について記載します。
まず、画面左上にある「Google APIs」をクリックし、
画面左にメニューペインを表示
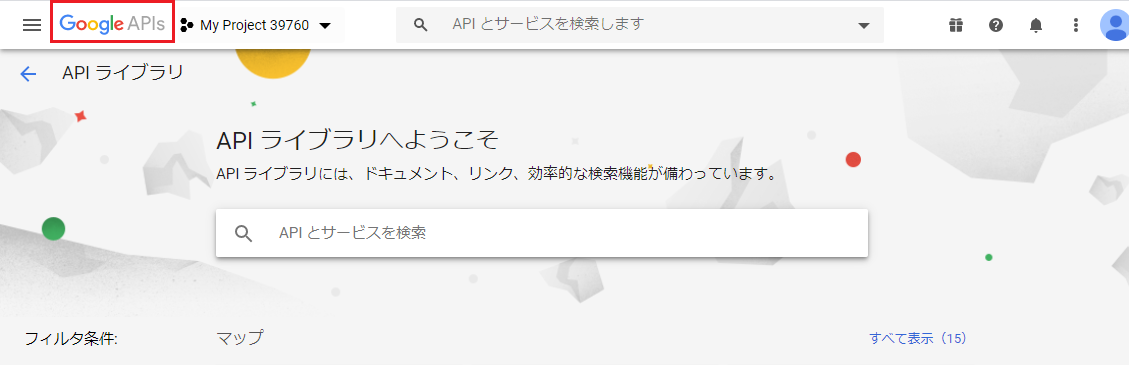
「認証情報」→「同意画面を構成」をクリック
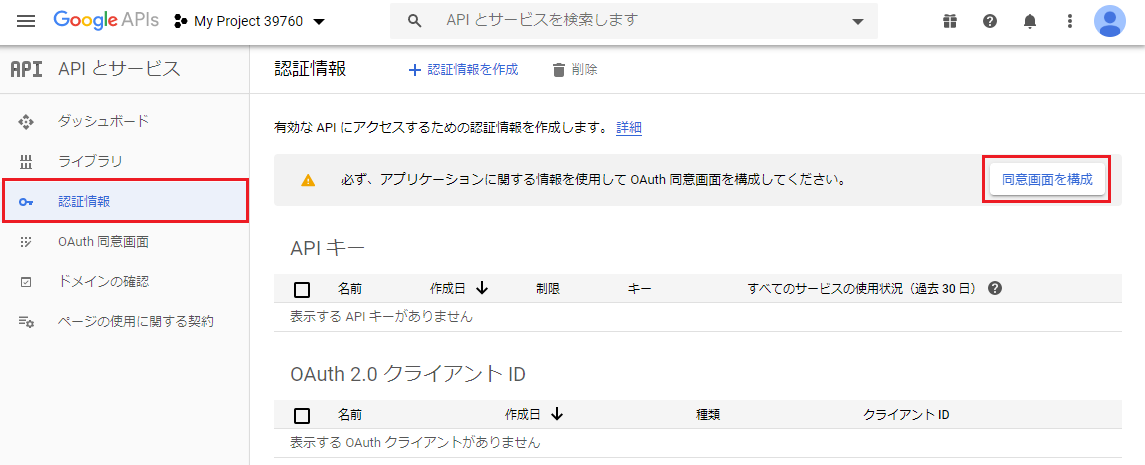
「OAuth同意画面」の「User Type」で「外部」を選択
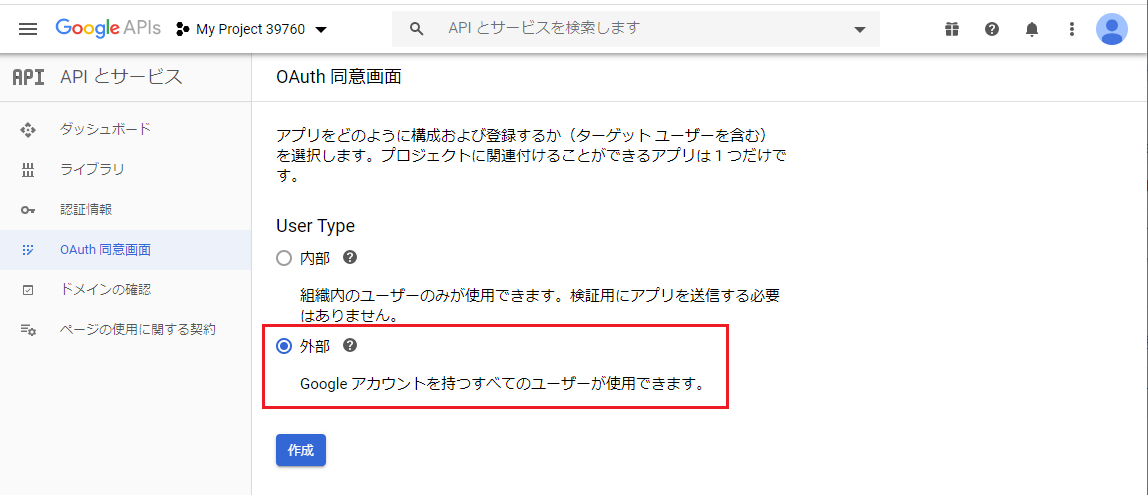
「OAuth同意画面」の「アプリケーション名」を入力。
(サポートメールは入力不要かもしれません)
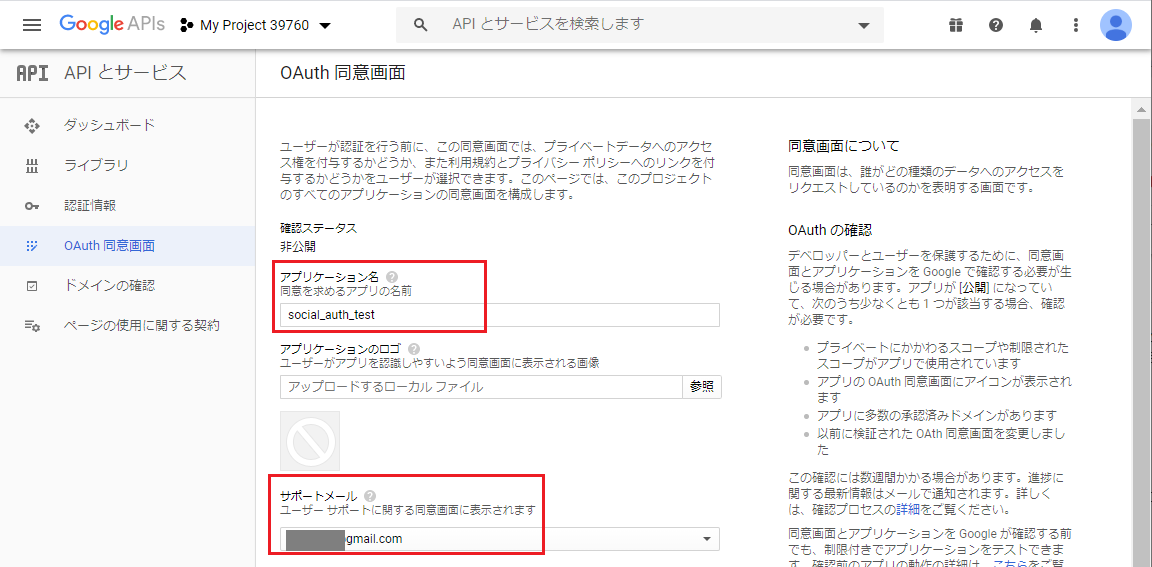
「認証情報を作成」→「OAuthクライアントID」をクリック
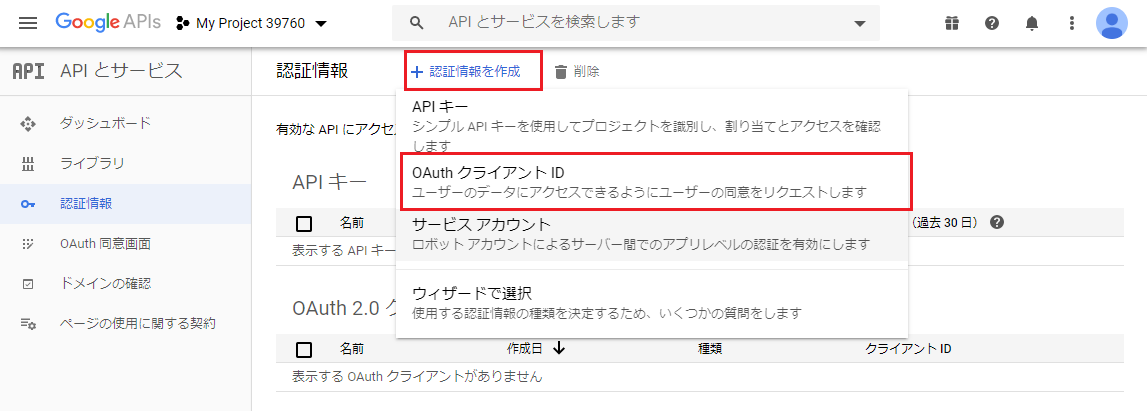
「OAuthクライアントの作成」において
「アプリケーションの種類」「名前」「承認済のリダイレクトURL」を入力
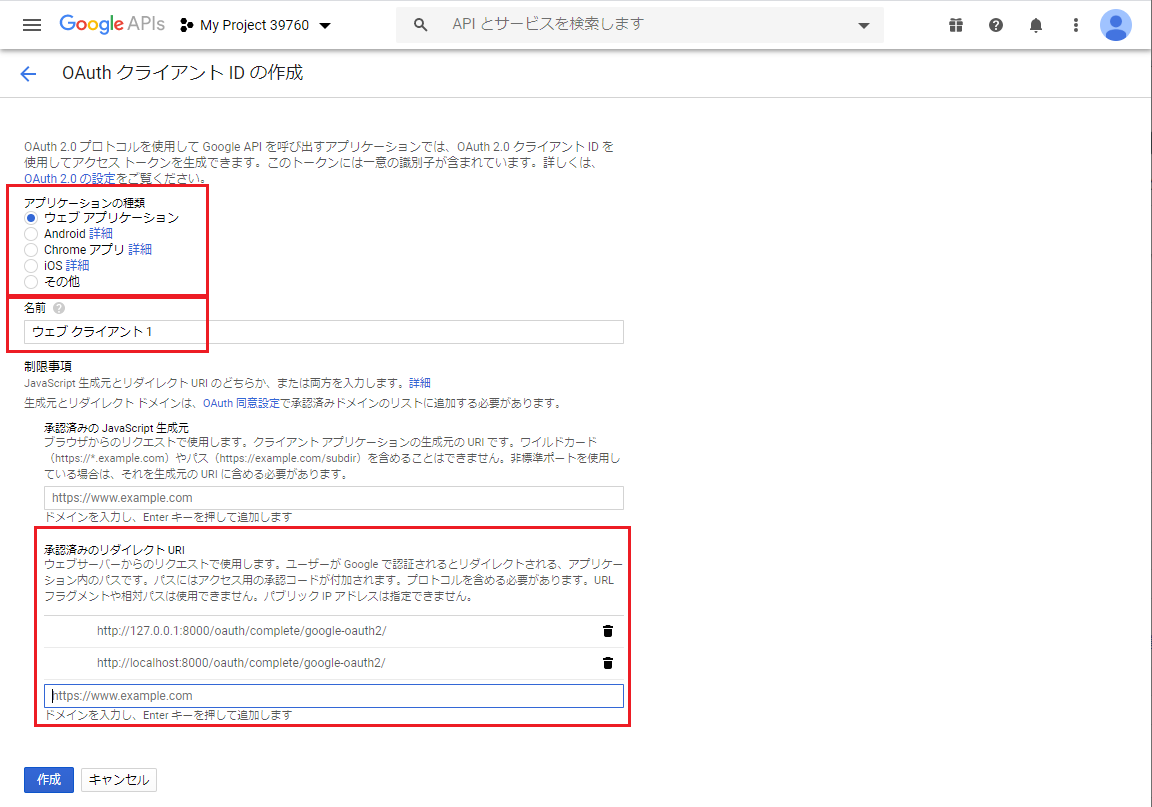
※私の場合、VirturlBox内のcentos8で動作させていることもあり
「承認済のリダイレクトURL」には以下を入力しています。
以上で、クライアント ID とシークレットが発行される為、
これを config/setting.py へ登録
$ vi config/setting.py
SOCIAL_AUTH_GOOGLE_OAUTH2_KEY = 'ないしょ' ## ADD
SOCIAL_AUTH_GOOGLE_OAUTH2_SECRET = 'ないしょ' ## ADD
Step5: view や template の作成
$ vi config/views.py ## NEW FILE
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
htmlテンプレートは、以下のurlにあるものをそのままcopyしました。
https://github.com/mila411/django-social-auth
Step6: django の起動と動作確認
最後に以下でdjango を起動すれば、ブラウザで動作確認可能です。
$ python manage.py runserver 0.0.0.0:8080